Akash and Vishal are quite fond of traveling. They mostly travel by railways. They were traveling on a train one day and they got interested in the seating arrangement of their compartment. The compartment looked something like
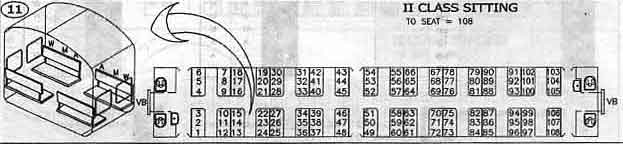
So they got interested to know the seat number facing them and the seat type facing them. The seats are denoted as follows:
- Window Seat: WS
- Middle Seat: MS
- Aisle Seat: AS
You will be given a seat number, find out the seat number facing you and the seat type, i.e. WS, MS or AS.
INPUT
The first line of input will consist of a single integer T denoting number of test-cases. Each test-case consists of a single integer N denoting the seat-number.
OUTPUT
For each test case, print the facing seat-number and the seat-type, separated by a single space in a new line.
CONSTRAINTS
PLEASE DO TRY SOLVING BY YOURSELF BEFORE READING THE WHOLE SOLUTION.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
|
#include <iostream>
using namespace std;
int main()
{
int t,n,rem=0;
cin>>t;
while(t--){
cin>>n;
rem = n%12;
switch(rem){
case 0:
cout<<(n-11)<<" WS"<<endl;
break;
case 1:
cout<<(n+11)<<" WS"<<endl;
break;
case 2:
cout<<(n+9)<<" MS"<<endl;
break;
case 3:
cout<<(n+7)<<" AS"<<endl;
break;
case 4:
cout<<(n+5)<<" AS"<<endl;
break;
case 5:
cout<<(n+3)<<" MS"<<endl;
break;
case 6:
cout<<(n+1)<<" WS"<<endl;
break;
case 7:
cout<<(n-1)<<" WS"<<endl;
break;
case 8:
cout<<(n-3)<<" MS"<<endl;
break;
case 9:
cout<<(n-5)<<" AS"<<endl;
break;
case 10:
cout<<(n-7)<<" AS"<<endl;
break;
case 11:
cout<<(n-9)<<" MS"<<endl;
break;
}
}
return 0;
}
|